Master scalable Flutter BLoC pattern architecture in 2025 with our comprehensive Flutter BLoC tutorial. Learn feature-based folder structure, clean separation of concerns, and state management techniques for building robust, maintainable applications.
Introduction
Building scalable mobile applications has become increasingly essential in today’s fast-paced development landscape. As applications grow in complexity, having a robust architecture becomes crucial for maintainability, testability, and developer productivity. Flutter, Google’s UI toolkit for building natively compiled applications, offers flexibility in implementing various architectural patterns. Among these, the Business Logic Component (BLoC) pattern stands out as a powerful solution for managing state and separating concerns in Flutter applications.
This comprehensive guide explores how to implement a feature-based folder structure with the BLoC pattern to create highly maintainable and scalable Flutter applications. We’ll dive deep into practical implementations, best practices, and real-world examples that you can apply to your projects immediately.
Understanding the BLoC Pattern in Flutter
The Core Concept of BLoC
The Business Logic Component (BLoC) pattern was introduced by Google engineers as a way to separate business logic from the UI layer. At its core, BLoC works on the principle of reactive programming, using streams to communicate between different layers of the application.
The fundamental BLoC architecture consists of three key components:
- Events: Actions or triggers sent to the BLoC from the UI
- States: Representations of the application’s condition at a specific point in time
- BLoC: The component that converts events into states
This stream-based approach offers several advantages:
- Unidirectional Data Flow: Data flows in a single direction, making the application more predictable
- Separation of Concerns: UI components remain focused on presentation while business logic stays in the BLoC
- Testability: Business logic can be tested independently of the UI
- Reusability: BLoCs can be reused across different UI components
BLoC vs. Other State Management Solutions
Flutter offers several state management solutions, including Provider, Redux, GetX, and Riverpod. The BLoC pattern distinguishes itself through:
- Structured Approach: BLoC enforces a clear structure for handling events and states
- Scalability: The pattern scales well for complex applications with numerous features
- Reactive Programming: Built on Dart’s stream capabilities for reactive updates
- Strong Community Support: Extensive documentation and community resources
While solutions like Provider might be simpler for small applications, BLoC shines in large-scale projects where clear separation of concerns becomes critical.
Setting Up a Feature-Based Folder Structure
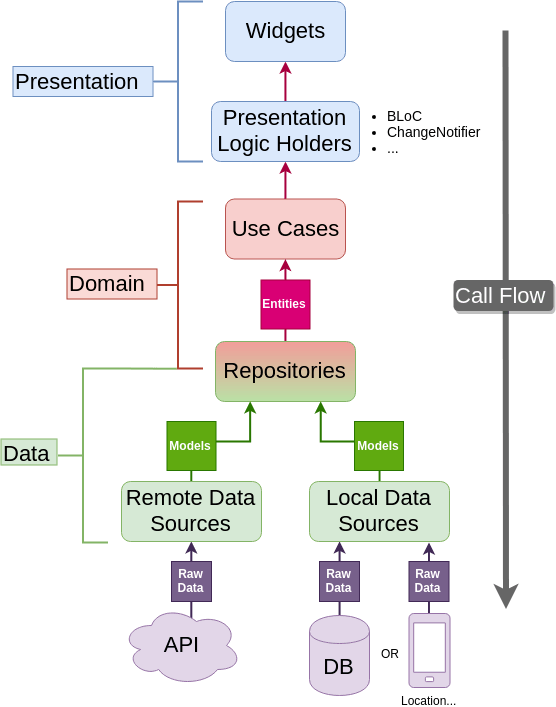
Why Choose a Feature-Based Approach?
Traditional folder structures often organize code by technical type (models, views, controllers), which becomes unwieldy as applications grow. A feature-based structure organizes code around business capabilities, offering:
- Improved Discoverability: Developers can quickly locate relevant code for a specific feature
- Encapsulation: Features remain self-contained with minimal dependencies
- Scalability: New features can be added without affecting existing code
- Team Collaboration: Different teams can work on separate features with minimal conflicts
Basic Feature-Based Structure Blueprint
A well-organized feature-based structure for a Flutter application using BLoC might look like this:
lib/
├── core/
│ ├── config/
│ ├── constants/
│ ├── errors/
│ ├── network/
│ ├── services/
│ ├── theme/
│ └── utils/
├── features/
│ ├── authentication/
│ │ ├── data/
│ │ │ ├── datasources/
│ │ │ ├── models/
│ │ │ └── repositories/
│ │ ├── domain/
│ │ │ ├── entities/
│ │ │ ├── repositories/
│ │ │ └── usecases/
│ │ └── presentation/
│ │ ├── bloc/
│ │ ├── pages/
│ │ └── widgets/
│ ├── home/
│ ├── profile/
│ └── settings/
├── main.dart
└── app.dart
This structure separates shared code in the core
directory from feature-specific code in the features
directory. Each feature follows a clean architecture approach with data, domain, and presentation layers.
Core Directory: The Application Foundation
The core
directory contains code that’s shared across features:
- Config: Application-wide configuration settings
- Constants: Global constants and string resources
- Errors: Custom error handling and exception classes
- Network: API clients and network utilities
- Services: Service locators and dependency injection setup
- Theme: Application theme configuration
- Utils: Helper functions and utility classes
Having a well-structured core provides a solid foundation for all features while avoiding code duplication.
Implementing Clean Architecture with BLoC
The Three Layers of Clean Architecture
To maximize the benefits with Flutter Architecture with BLoC, we’ll implement it within a clean architecture framework, dividing each feature into three layers:
- Presentation Layer: UI components and BLoCs
- Domain Layer: Business rules, entities, and use cases
- Data Layer: Data sources and repositories
This layered approach ensures that business logic remains independent of the framework and external dependencies.
Data Layer: Handling External Data
The data layer is responsible for retrieving and storing data. It consists of:
- Data Sources: Classes that handle API calls or local database operations
- Models: Data classes that map to external data structures
- Repositories Implementations: Concrete implementations of repository interfaces
Here’s an example of a repository implementation for a cryptocurrency feature:
class CryptoRepositoryImpl implements CryptoRepository {
final CryptoRemoteDataSource remoteDataSource;
final NetworkInfo networkInfo;
CryptoRepositoryImpl({
required this.remoteDataSource,
required this.networkInfo,
});
@override
Future<Either<Failure, List<Cryptocurrency>>> getTopCryptocurrencies() async {
if (await networkInfo.isConnected) {
try {
final remoteCryptos = await remoteDataSource.getTopCryptocurrencies();
return Right(remoteCryptos);
} on ServerException {
return Left(ServerFailure());
}
} else {
return Left(NetworkFailure());
}
}
}
Domain Layer: Business Logic Central
The domain layer encapsulates the core business logic and consists of:
- Entities: Core business objects
- Repositories: Interfaces defining data operations
- Use Cases: Single-responsibility classes for business operations
A use case for fetching cryptocurrency data might look like:
class GetTopCryptocurrencies {
final CryptoRepository repository;
GetTopCryptocurrencies(this.repository);
Future<Either<Failure, List<Cryptocurrency>>> execute() {
return repository.getTopCryptocurrencies();
}
}
Presentation Layer: Bringing BLoC Into Play
The presentation layer is where the BLoC pattern truly shines. It includes:
- BLoC: Classes converting events to states
- Events: Classes representing UI actions
- States: Classes representing UI states
- Pages: Screen-level UI components
- Widgets: Reusable UI components
For a cryptocurrency feature, we might define:
// Event
abstract class CryptoEvent extends Equatable {
@override
List<Object> get props => [];
}
class FetchCryptocurrencies extends CryptoEvent {}
// State
abstract class CryptoState extends Equatable {
@override
List<Object> get props => [];
}
class CryptoInitial extends CryptoState {}
class CryptoLoading extends CryptoState {}
class CryptoLoaded extends CryptoState {
final List<Cryptocurrency> cryptocurrencies;
CryptoLoaded(this.cryptocurrencies);
@override
List<Object> get props => [cryptocurrencies];
}
class CryptoError extends CryptoState {
final String message;
CryptoError(this.message);
@override
List<Object> get props => [message];
}
// BLoC
class CryptoBloc extends Bloc<CryptoEvent, CryptoState> {
final GetTopCryptocurrencies getTopCryptocurrencies;
CryptoBloc({required this.getTopCryptocurrencies}) : super(CryptoInitial()) {
on<FetchCryptocurrencies>(_onFetchCryptocurrencies);
}
Future<void> _onFetchCryptocurrencies(
FetchCryptocurrencies event,
Emitter<CryptoState> emit,
) async {
emit(CryptoLoading());
final result = await getTopCryptocurrencies.execute();
result.fold(
(failure) => emit(CryptoError(_mapFailureToMessage(failure))),
(cryptocurrencies) => emit(CryptoLoaded(cryptocurrencies)),
);
}
String _mapFailureToMessage(Failure failure) {
// Map different failures to user-friendly messages
switch (failure.runtimeType) {
case ServerFailure:
return 'Server error occurred';
case NetworkFailure:
return 'Please check your internet connection';
default:
return 'Unexpected error';
}
}
}
Connecting the Layers with Dependency Injection
The Importance of Dependency Injection
To maintain separation between layers, we need a dependency injection system. This allows:
- Inversion of Control: Higher-level modules don’t depend on lower-level modules
- Testability: Dependencies can be easily mocked during tests
- Flexibility: Implementations can be swapped without changing dependent code
Setting Up GetIt for Dependency Injection
GetIt is a lightweight service locator that’s perfect for Flutter applications:
final GetIt sl = GetIt.instance;
void initializeDependencies() {
// BLoCs
sl.registerFactory(
() => CryptoBloc(getTopCryptocurrencies: sl()),
);
// Use Cases
sl.registerLazySingleton(() => GetTopCryptocurrencies(sl()));
// Repositories
sl.registerLazySingleton<CryptoRepository>(
() => CryptoRepositoryImpl(
remoteDataSource: sl(),
networkInfo: sl(),
),
);
// Data Sources
sl.registerLazySingleton<CryptoRemoteDataSource>(
() => CryptoRemoteDataSourceImpl(client: sl()),
);
// Core
sl.registerLazySingleton<NetworkInfo>(
() => NetworkInfoImpl(sl()),
);
// External
sl.registerLazySingleton(() => http.Client());
sl.registerLazySingleton(() => InternetConnectionChecker());
}
Real-World Implementation: Cryptocurrency Tracking App
Setting Up the Project
Let’s apply the architecture to build a cryptocurrency tracking application for FlutterCoinHub. Start by creating a new Flutter project:
flutter create flutter_coin_hub
cd flutter_coin_hub
Add the necessary dependencies to pubspec.yaml
:
dependencies:
flutter:
sdk: flutter
# State management
flutter_bloc: ^8.1.1
equatable: ^2.0.5
# Networking
http: ^0.13.5
internet_connection_checker: ^1.0.0
# Dependency injection
get_it: ^7.2.0
# Functional programming
dartz: ^0.10.1
# Local storage
shared_preferences: ^2.0.17
Implementing Core Components
First, let’s set up some core components:
Network Info:
// lib/core/network/network_info.dart
abstract class NetworkInfo {
Future<bool> get isConnected;
}
class NetworkInfoImpl implements NetworkInfo {
final InternetConnectionChecker connectionChecker;
NetworkInfoImpl(this.connectionChecker);
@override
Future<bool> get isConnected => connectionChecker.hasConnection;
}
Error Handling:
// lib/core/errors/failures.dart
abstract class Failure extends Equatable {
@override
List<Object> get props => [];
}
class ServerFailure extends Failure {}
class NetworkFailure extends Failure {}
// lib/core/errors/exceptions.dart
class ServerException implements Exception {}
class CacheException implements Exception {}
Building the Cryptocurrency Feature
Now let’s implement the cryptocurrency feature following our architecture:
Domain Layer:
// lib/features/cryptocurrency/domain/entities/cryptocurrency.dart
class Cryptocurrency extends Equatable {
final String id;
final String name;
final String symbol;
final double price;
final double changePercentage24h;
final double marketCap;
const Cryptocurrency({
required this.id,
required this.name,
required this.symbol,
required this.price,
required this.changePercentage24h,
required this.marketCap,
});
@override
List<Object> get props => [id, name, symbol, price, changePercentage24h, marketCap];
}
// lib/features/cryptocurrency/domain/repositories/crypto_repository.dart
abstract class CryptoRepository {
Future<Either<Failure, List<Cryptocurrency>>> getTopCryptocurrencies();
Future<Either<Failure, Cryptocurrency>> getCryptocurrencyDetails(String id);
}
// lib/features/cryptocurrency/domain/usecases/get_top_cryptocurrencies.dart
class GetTopCryptocurrencies {
final CryptoRepository repository;
GetTopCryptocurrencies(this.repository);
Future<Either<Failure, List<Cryptocurrency>>> execute() {
return repository.getTopCryptocurrencies();
}
}
Data Layer:
// lib/features/cryptocurrency/data/models/cryptocurrency_model.dart
class CryptocurrencyModel extends Cryptocurrency {
const CryptocurrencyModel({
required String id,
required String name,
required String symbol,
required double price,
required double changePercentage24h,
required double marketCap,
}) : super(
id: id,
name: name,
symbol: symbol,
price: price,
changePercentage24h: changePercentage24h,
marketCap: marketCap,
);
factory CryptocurrencyModel.fromJson(Map<String, dynamic> json) {
return CryptocurrencyModel(
id: json['id'],
name: json['name'],
symbol: json['symbol'],
price: (json['current_price'] as num).toDouble(),
changePercentage24h: (json['price_change_percentage_24h'] as num).toDouble(),
marketCap: (json['market_cap'] as num).toDouble(),
);
}
Map<String, dynamic> toJson() {
return {
'id': id,
'name': name,
'symbol': symbol,
'current_price': price,
'price_change_percentage_24h': changePercentage24h,
'market_cap': marketCap,
};
}
}
// lib/features/cryptocurrency/data/datasources/crypto_remote_data_source.dart
abstract class CryptoRemoteDataSource {
Future<List<CryptocurrencyModel>> getTopCryptocurrencies();
Future<CryptocurrencyModel> getCryptocurrencyDetails(String id);
}
class CryptoRemoteDataSourceImpl implements CryptoRemoteDataSource {
final http.Client client;
final String baseUrl = 'https://api.coingecko.com/api/v3';
CryptoRemoteDataSourceImpl({required this.client});
@override
Future<List<CryptocurrencyModel>> getTopCryptocurrencies() async {
final response = await client.get(
Uri.parse('$baseUrl/coins/markets?vs_currency=usd&order=market_cap_desc&per_page=100'),
headers: {'Content-Type': 'application/json'},
);
if (response.statusCode == 200) {
final List<dynamic> jsonList = json.decode(response.body);
return jsonList.map((json) => CryptocurrencyModel.fromJson(json)).toList();
} else {
throw ServerException();
}
}
@override
Future<CryptocurrencyModel> getCryptocurrencyDetails(String id) async {
final response = await client.get(
Uri.parse('$baseUrl/coins/$id'),
headers: {'Content-Type': 'application/json'},
);
if (response.statusCode == 200) {
return CryptocurrencyModel.fromJson(json.decode(response.body));
} else {
throw ServerException();
}
}
}
// lib/features/cryptocurrency/data/repositories/crypto_repository_impl.dart
class CryptoRepositoryImpl implements CryptoRepository {
final CryptoRemoteDataSource remoteDataSource;
final NetworkInfo networkInfo;
CryptoRepositoryImpl({
required this.remoteDataSource,
required this.networkInfo,
});
@override
Future<Either<Failure, List<Cryptocurrency>>> getTopCryptocurrencies() async {
if (await networkInfo.isConnected) {
try {
final remoteCryptos = await remoteDataSource.getTopCryptocurrencies();
return Right(remoteCryptos);
} on ServerException {
return Left(ServerFailure());
}
} else {
return Left(NetworkFailure());
}
}
@override
Future<Either<Failure, Cryptocurrency>> getCryptocurrencyDetails(String id) async {
if (await networkInfo.isConnected) {
try {
final remoteCrypto = await remoteDataSource.getCryptocurrencyDetails(id);
return Right(remoteCrypto);
} on ServerException {
return Left(ServerFailure());
}
} else {
return Left(NetworkFailure());
}
}
}
Presentation Layer:
// lib/features/cryptocurrency/presentation/bloc/crypto_bloc.dart
class CryptoBloc extends Bloc<CryptoEvent, CryptoState> {
final GetTopCryptocurrencies getTopCryptocurrencies;
CryptoBloc({required this.getTopCryptocurrencies}) : super(CryptoInitial()) {
on<FetchCryptocurrencies>(_onFetchCryptocurrencies);
}
Future<void> _onFetchCryptocurrencies(
FetchCryptocurrencies event,
Emitter<CryptoState> emit,
) async {
emit(CryptoLoading());
final result = await getTopCryptocurrencies.execute();
result.fold(
(failure) => emit(CryptoError(_mapFailureToMessage(failure))),
(cryptocurrencies) => emit(CryptoLoaded(cryptocurrencies)),
);
}
String _mapFailureToMessage(Failure failure) {
switch (failure.runtimeType) {
case ServerFailure:
return 'Server error occurred';
case NetworkFailure:
return 'Please check your internet connection';
default:
return 'Unexpected error';
}
}
}
// lib/features/cryptocurrency/presentation/pages/cryptocurrency_list_page.dart
class CryptocurrencyListPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('FlutterCoinHub'),
),
body: BlocProvider(
create: (_) => sl<CryptoBloc>()..add(FetchCryptocurrencies()),
child: BlocBuilder<CryptoBloc, CryptoState>(
builder: (context, state) {
if (state is CryptoInitial) {
return Center(child: Text('Start exploring cryptocurrencies'));
} else if (state is CryptoLoading) {
return Center(child: CircularProgressIndicator());
} else if (state is CryptoLoaded) {
return CryptocurrencyListView(
cryptocurrencies: state.cryptocurrencies,
);
} else if (state is CryptoError) {
return Center(child: Text(state.message));
} else {
return Center(child: Text('Something went wrong'));
}
},
),
),
);
}
}
// lib/features/cryptocurrency/presentation/widgets/cryptocurrency_list_view.dart
class CryptocurrencyListView extends StatelessWidget {
final List<Cryptocurrency> cryptocurrencies;
const CryptocurrencyListView({
Key? key,
required this.cryptocurrencies,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: cryptocurrencies.length,
itemBuilder: (context, index) {
final crypto = cryptocurrencies[index];
return CryptocurrencyListTile(cryptocurrency: crypto);
},
);
}
}
// lib/features/cryptocurrency/presentation/widgets/cryptocurrency_list_tile.dart
class CryptocurrencyListTile extends StatelessWidget {
final Cryptocurrency cryptocurrency;
const CryptocurrencyListTile({
Key? key,
required this.cryptocurrency,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return ListTile(
leading: CircleAvatar(
child: Text(cryptocurrency.symbol.toUpperCase().substring(0, 1)),
),
title: Text(cryptocurrency.name),
subtitle: Text(cryptocurrency.symbol.toUpperCase()),
trailing: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.end,
children: [
Text(
'\$${cryptocurrency.price.toStringAsFixed(2)}',
style: TextStyle(fontWeight: FontWeight.bold),
),
Text(
'${cryptocurrency.changePercentage24h >= 0 ? '+' : ''}${cryptocurrency.changePercentage24h.toStringAsFixed(2)}%',
style: TextStyle(
color: cryptocurrency.changePercentage24h >= 0
? Colors.green
: Colors.red,
),
),
],
),
onTap: () {
// Navigate to detail page
},
);
}
}
Advanced BLoC Patterns and Techniques
Handling Multiple Events and States
As applications grow, BLoCs may need to handle multiple related events and states. Consider a more complex cryptocurrency detail page that displays price history along with general information:
// Events
abstract class CryptoDetailEvent extends Equatable {
@override
List<Object> get props => [];
}
class FetchCryptoDetail extends CryptoDetailEvent {
final String cryptoId;
FetchCryptoDetail(this.cryptoId);
@override
List<Object> get props => [cryptoId];
}
class FetchCryptoPriceHistory extends CryptoDetailEvent {
final String cryptoId;
final String timeFrame; // e.g., '1d', '7d', '30d'
FetchCryptoPriceHistory(this.cryptoId, this.timeFrame);
@override
List<Object> get props => [cryptoId, timeFrame];
}
// States
abstract class CryptoDetailState extends Equatable {
@override
List<Object> get props => [];
}
class CryptoDetailInitial extends CryptoDetailState {}
class CryptoDetailLoading extends CryptoDetailState {}
class CryptoDetailLoaded extends CryptoDetailState {
final Cryptocurrency cryptocurrency;
CryptoDetailLoaded(this.cryptocurrency);
@override
List<Object> get props => [cryptocurrency];
}
class CryptoPriceHistoryLoading extends CryptoDetailState {}
class CryptoPriceHistoryLoaded extends CryptoDetailState {
final List<PricePoint> priceHistory;
CryptoPriceHistoryLoaded(this.priceHistory);
@override
List<Object> get props => [priceHistory];
}
class CryptoDetailError extends CryptoDetailState {
final String message;
CryptoDetailError(this.message);
@override
List<Object> get props => [message];
}
// BLoC
class CryptoDetailBloc extends Bloc<CryptoDetailEvent, CryptoDetailState> {
final GetCryptocurrencyDetail getCryptocurrencyDetail;
final GetCryptocurrencyPriceHistory getCryptocurrencyPriceHistory;
CryptoDetailBloc({
required this.getCryptocurrencyDetail,
required this.getCryptocurrencyPriceHistory,
}) : super(CryptoDetailInitial()) {
on<FetchCryptoDetail>(_onFetchCryptoDetail);
on<FetchCryptoPriceHistory>(_onFetchCryptoPriceHistory);
}
Future<void> _onFetchCryptoDetail(
FetchCryptoDetail event,
Emitter<CryptoDetailState> emit,
) async {
emit(CryptoDetailLoading());
final result = await getCryptocurrencyDetail.execute(event.cryptoId);
result.fold(
(failure) => emit(CryptoDetailError(_mapFailureToMessage(failure))),
(cryptocurrency) => emit(CryptoDetailLoaded(cryptocurrency)),
);
}
Future<void> _onFetchCryptoPriceHistory(
FetchCryptoPriceHistory event,
Emitter<CryptoDetailState> emit,
) async {
emit(CryptoPriceHistoryLoading());
final result = await getCryptocurrencyPriceHistory.execute(
event.cryptoId,
event.timeFrame,
);
result.fold(
(failure) => emit(CryptoDetailError(_mapFailureToMessage(failure))),
(priceHistory) => emit(CryptoPriceHistoryLoaded(priceHistory)),
);
}
String _mapFailureToMessage(Failure failure) {
// Implementation similar to previous example
}
}
BLoC to BLoC Communication
Sometimes BLoCs need to communicate with each other. This can be achieved using streams or through a shared service:
// Using StreamSubscription
class WatchlistBloc extends Bloc<WatchlistEvent, WatchlistState> {
final CryptoBloc cryptoBloc;
late final StreamSubscription cryptoSubscription;
WatchlistBloc({required this.cryptoBloc}) : super(WatchlistInitial()) {
on<AddToWatchlist>(_onAddToWatchlist);
on<RemoveFromWatchlist>(_onRemoveFromWatchlist);
on<UpdateWatchlistPrices>(_onUpdateWatchlistPrices);
cryptoSubscription = cryptoBloc.stream.listen((state) {
if (state is CryptoLoaded) {
add(UpdateWatchlistPrices(state.cryptocurrencies));
}
});
}
@override
Future<void> close() {
cryptoSubscription.cancel();
return super.close();
}
// Event handlers implementation
}
Error Handling and Recovery
Robust error handling is crucial for a good user experience. We can enhance our BLoCs to handle different types of errors and provide recovery mechanisms:
class CryptoBloc extends Bloc<CryptoEvent, CryptoState> {
final GetTopCryptocurrencies getTopCryptocurrencies;
CryptoBloc({required this.getTopCryptocurrencies}) : super(CryptoInitial()) {
on<FetchCryptocurrencies>(_onFetchCryptocurrencies);
on<RetryFetchCryptocurrencies>(_onRetryFetchCryptocurrencies);
}
Future<void> _onFetchCryptocurrencies(
FetchCryptocurrencies event,
Emitter<CryptoState> emit,
) async {
emit(CryptoLoading());
await _fetchCryptocurrencies(emit);
}
Future<void> _onRetryFetchCryptocurrencies(
RetryFetchCryptocurrencies event,
Emitter<CryptoState> emit,
) async {
emit(CryptoLoading());
await _fetchCryptocurrencies(emit);
}
Future<void> _fetchCryptocurrencies(Emitter<CryptoState> emit) async {
final result = await getTopCryptocurrencies.execute();
result.fold(
(failure) => emit(CryptoError(
message: _mapFailureToMessage(failure),
failure: failure,
)),
(cryptocurrencies) => emit(CryptoLoaded(cryptocurrencies)),
);
}
}
On the UI side, we can provide a retry button:
BlocBuilder<CryptoBloc, CryptoState>(
builder: (context, state) {
if (state is CryptoError) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(state.message),
SizedBox(height: 16),
ElevatedButton(
onPressed: () {
context.read<CryptoBloc>().add(RetryFetchCryptocurrencies());
},
child: Text('Retry'),
),
],
);
}
// Other state handling
},
)
Testing Your BLoC Architecture
Unit Testing BLoCs
One of the major advantages of the BLoC pattern is testability. Here’s how to unit test a BLoC:
void main() {
late CryptoBloc cryptoBloc;
late MockGetTopCryptocurrencies mockGetTopCryptocurrencies;
setUp(() {
mockGetTopCryptocurrencies = MockGetTopCryptocurrencies();
cryptoBloc = CryptoBloc(getTopCryptocurrencies: mockGetTopCryptocurrencies);
});
tearDown(() {
cryptoBloc.close();
});
test('initial state should be CryptoInitial', () {
// assert
expect(cryptoBloc.state, equals(CryptoInitial()));
});
group('FetchCryptocurrencies', () {
final tCryptocurrencies = [
Cryptocurrency(
id: 'bitcoin',
name: 'Bitcoin',
symbol: 'btc',
price: 50000.0,
changePercentage24h: 2.5,
marketCap: 1000000000.0,
),
];
test(
'should emit [CryptoLoading, CryptoLoaded] when data is fetched successfully',
() async {
// arrange
when(mockGetTopCryptocurrencies.execute())
.thenAnswer((_) async => Right(tCryptocurrencies));
// assert later
final expected = [
CryptoLoading(),
CryptoLoaded(tCryptocurrencies),
];
expectLater(cryptoBloc.stream.asBroadcastStream(), emitsInOrder(expected));
// act
cryptoBloc.add(FetchCryptocurrencies());
},
);
test(
'should emit [CryptoLoading, CryptoError] when getting data fails',
() async {
// arrange
when(mockGetTopCryptocurrencies.execute())
.thenAnswer((_) async => Left(ServerFailure()));
// assert later
final expected = [
CryptoLoading(),
CryptoError('Server error occurred'),
];
expectLater(cryptoBloc.stream.asBroadcastStream(), emitsInOrder(expected));
// act
cryptoBloc.add(FetchCryptocurrencies());
},
);
});
}
Widget Testing with BLoC
Widget testing ensures your UI components work correctly with BLoCs:
Conclusion
Implementing a scalable Flutter architecture with the BLoC pattern provides a solid foundation for applications of any size. By separating business logic from the UI layer, the BLoC pattern enables better testability, maintainability, and scalability.
In this comprehensive guide, we’ve covered everything from basic BLoC implementation to advanced techniques for real-world applications. By following these principles and best practices, you can build Flutter applications that are not only beautiful but also robust and maintainable as they grow in complexity.
Remember that architecture is not one-size-fits-all. The BLoC pattern offers flexibility, allowing you to adapt it to your specific project requirements. As your application evolves, continuously evaluate and refine your architecture to ensure it remains effective and scalable.
Happy coding with Flutter and BLoC!